Popup:
In this blog, I will show you how to open new form as pop up when clicked on a ribbon workbench button.
First create a button using ribbon workbench
Code i have written for this session
Just replace the entityName with your targeted entity then it will work and width value is your.
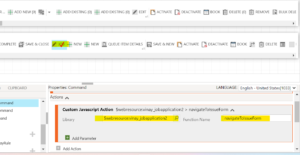
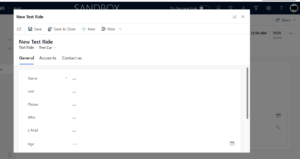
For any Help or Queries Contact us on info@crmonce.com or +918096556344
The CreatePass OnWorkerStatusApproved
plugin is a custom plugin developed for Microsoft Dynamics 365 (CRM). It triggers when the status of a worker entity (crm_worker
) is updated. Specifically, it runs when the worker’s status is changed to “Approved”. When triggered, the plugin automatically creates a related “Pass” entity (crm_pass
) with details pulled from the worker record.
using System;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Query;
public class CreatePassOnWorkerStatusApproved : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
ITracingService tracingService = (ITracingService)serviceProvider.GetService(typeof(ITracingService));
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: Execution started.”);
try
{
if (context.MessageName != “Update”)
return;
tracingService.Trace(“Message is Update.”);
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
Entity entity = (Entity)context.InputParameters[“Target”];
if (entity.LogicalName == “crm_worker” && entity.Contains(“crm_workerstatus”))
{
tracingService.Trace(“Entity is crm_worker and contains workstatus.”);
Entity workerEntity = service.Retrieve(“crm_worker”, entity.Id, new ColumnSet(true));
tracingService.Trace(“Worker entity retrieved. Worker Id: {0}”, workerEntity.Id);
if (workerEntity.GetAttributeValue<OptionSetValue>(“crm_workerstatus”).Value == 770060001)
{
tracingService.Trace(“Worker status is Approved.”);
Entity passEntity = new Entity(“crm_pass”);
passEntity[“crm_name”] = workerEntity.GetAttributeValue<string>(“crm_passnumber”);
passEntity[“crm_passexpiredate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passexpiredate”);
passEntity[“crm_passissuedate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passissuedate”);
passEntity[“crm_department”] = workerEntity.GetAttributeValue<string>(“crm_department”);
passEntity[“crm_designation”] = workerEntity.GetAttributeValue<string>(“crm_designation”);
passEntity[“crm_worker”] = new EntityReference(“crm_worker”, workerEntity.Id);
tracingService.Trace(“crm_worker lookup set.”);
passEntity[“crm_passstatus”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passstatus”);
tracingService.Trace(“crm_passstatus set.”);
passEntity[“crm_passtype”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passtype”);
tracingService.Trace(“crm_passtype set.”);
if (workerEntity.Contains(“crm_companyname”))
{
EntityReference accountRef = workerEntity.GetAttributeValue<EntityReference>(“crm_companyname”);
Entity accountEntity = service.Retrieve(“crm_company”, accountRef.Id, new ColumnSet(“crm_name”));
passEntity[“crm_companyname”] = accountEntity.GetAttributeValue<string>(“crm_name”);
tracingService.Trace(“Account name set in crm_company field.”);
}
service.Create(passEntity);
tracingService.Trace(“Pass record created successfully.”);
}
}
}
catch (Exception ex)
{
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: {0}”, ex.ToString());
throw;
}
}
}
The CreatePass OnWorkerStatusApproved
plugin is a custom plugin developed for Microsoft Dynamics 365 (CRM). It triggers when the status of a worker entity (crm_worker
) is updated. Specifically, it runs when the worker’s status is changed to “Approved”. When triggered, the plugin automatically creates a related “Pass” entity (crm_pass
) with details pulled from the worker record.
using System;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Query;
public class CreatePassOnWorkerStatusApproved : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
ITracingService tracingService = (ITracingService)serviceProvider.GetService(typeof(ITracingService));
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: Execution started.”);
try
{
if (context.MessageName != “Update”)
return;
tracingService.Trace(“Message is Update.”);
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
Entity entity = (Entity)context.InputParameters[“Target”];
if (entity.LogicalName == “crm_worker” && entity.Contains(“crm_workerstatus”))
{
tracingService.Trace(“Entity is crm_worker and contains workstatus.”);
Entity workerEntity = service.Retrieve(“crm_worker”, entity.Id, new ColumnSet(true));
tracingService.Trace(“Worker entity retrieved. Worker Id: {0}”, workerEntity.Id);
if (workerEntity.GetAttributeValue<OptionSetValue>(“crm_workerstatus”).Value == 770060001)
{
tracingService.Trace(“Worker status is Approved.”);
Entity passEntity = new Entity(“crm_pass”);
passEntity[“crm_name”] = workerEntity.GetAttributeValue<string>(“crm_passnumber”);
passEntity[“crm_passexpiredate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passexpiredate”);
passEntity[“crm_passissuedate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passissuedate”);
passEntity[“crm_department”] = workerEntity.GetAttributeValue<string>(“crm_department”);
passEntity[“crm_designation”] = workerEntity.GetAttributeValue<string>(“crm_designation”);
passEntity[“crm_worker”] = new EntityReference(“crm_worker”, workerEntity.Id);
tracingService.Trace(“crm_worker lookup set.”);
passEntity[“crm_passstatus”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passstatus”);
tracingService.Trace(“crm_passstatus set.”);
passEntity[“crm_passtype”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passtype”);
tracingService.Trace(“crm_passtype set.”);
if (workerEntity.Contains(“crm_companyname”))
{
EntityReference accountRef = workerEntity.GetAttributeValue<EntityReference>(“crm_companyname”);
Entity accountEntity = service.Retrieve(“crm_company”, accountRef.Id, new ColumnSet(“crm_name”));
passEntity[“crm_companyname”] = accountEntity.GetAttributeValue<string>(“crm_name”);
tracingService.Trace(“Account name set in crm_company field.”);
}
service.Create(passEntity);
tracingService.Trace(“Pass record created successfully.”);
}
}
}
catch (Exception ex)
{
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: {0}”, ex.ToString());
throw;
}
}
}