Automatically Creates a Pass:
- Automatically new crm_pass entity is created and populated with data from the crm_worker record.
- Fields like crm_name, crm_passexpiredate, crm_passissuedate, crm_department, and crm_designation are copied over.
- The plugin also sets the crm_worker lookup on the crm_pass entity, linking it to the original worker record.
- OptionSet values like crm_passstatus and crm_passtype are also copied from the worker record.
- If the worker is associated with a company (crm_companyname), the plugin retrieves the company’s name and sets it in the crm_pass record.
- Pass Entity Creation: The newly created crm_pass entity is then saved to the CRM system.
Error Handling: If any exceptions occur during the process, they are caught, traced, and re-thrown to ensure proper logging and notification.
using System;
using Microsoft.Xrm.Sdk;
using Microsoft.Xrm.Sdk.Query;
public class CreatePassOnWorkerStatusApproved : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
ITracingService tracingService = (ITracingService)serviceProvider.GetService(typeof(ITracingService));
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: Execution started.”);
try
{
if (context.MessageName != “Update”)
return;
tracingService.Trace(“Message is Update.”);
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
Entity entity = (Entity)context.InputParameters[“Target”];
if (entity.LogicalName == “crm_worker” && entity.Contains(“crm_workerstatus”))
{
tracingService.Trace(“Entity is crm_worker and contains workstatus.”);
Entity workerEntity = service.Retrieve(“crm_worker”, entity.Id, new ColumnSet(true));
tracingService.Trace(“Worker entity retrieved. Worker Id: {0}”, workerEntity.Id);
if (workerEntity.GetAttributeValue<OptionSetValue>(“crm_workerstatus”).Value == 770060001)
{
tracingService.Trace(“Worker status is Approved.”);
Entity passEntity = new Entity(“crm_pass”);
passEntity[“crm_name”] = workerEntity.GetAttributeValue<string>(“crm_passnumber”);
passEntity[“crm_passexpiredate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passexpiredate”);
passEntity[“crm_passissuedate”] = workerEntity.GetAttributeValue<DateTime>(“crm_passissuedate”);
passEntity[“crm_department”] = workerEntity.GetAttributeValue<string>(“crm_department”);
passEntity[“crm_designation”] = workerEntity.GetAttributeValue<string>(“crm_designation”);
passEntity[“crm_worker”] = new EntityReference(“crm_worker”, workerEntity.Id);
tracingService.Trace(“crm_worker lookup set.”);
passEntity[“crm_passstatus”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passstatus”);
tracingService.Trace(“crm_passstatus set.”);
passEntity[“crm_passtype”] = workerEntity.GetAttributeValue<OptionSetValue>(“crm_passtype”);
tracingService.Trace(“crm_passtype set.”);
if (workerEntity.Contains(“crm_companyname”))
{
EntityReference accountRef = workerEntity.GetAttributeValue<EntityReference>(“crm_companyname”);
Entity accountEntity = service.Retrieve(“crm_company”, accountRef.Id, new ColumnSet(“crm_name”));
passEntity[“crm_companyname”] = accountEntity.GetAttributeValue<string>(“crm_name”);
tracingService.Trace(“Account name set in crm_company field.”);
}
service.Create(passEntity);
tracingService.Trace(“Pass record created successfully.”);
}
}
}
catch (Exception ex)
{
tracingService.Trace(“CreatePassOnWorkerStatusApproved Plugin: {0}”, ex.ToString());
throw;
}
}
}
Here, the worker status is pending approval; the worker pass has not yet been generated.
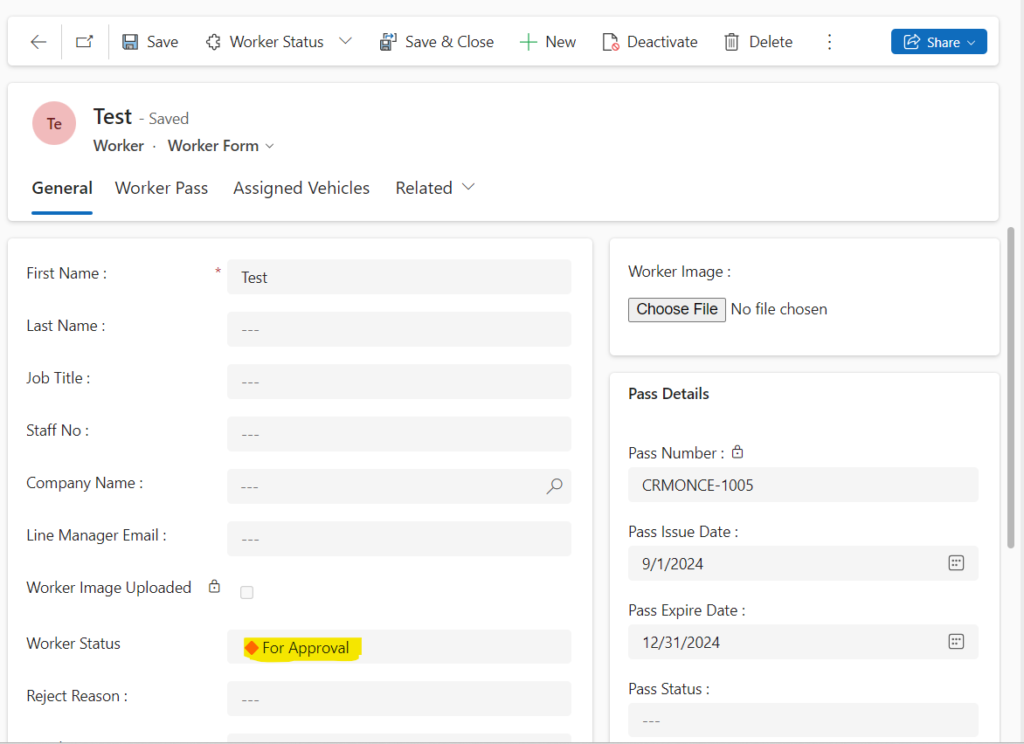
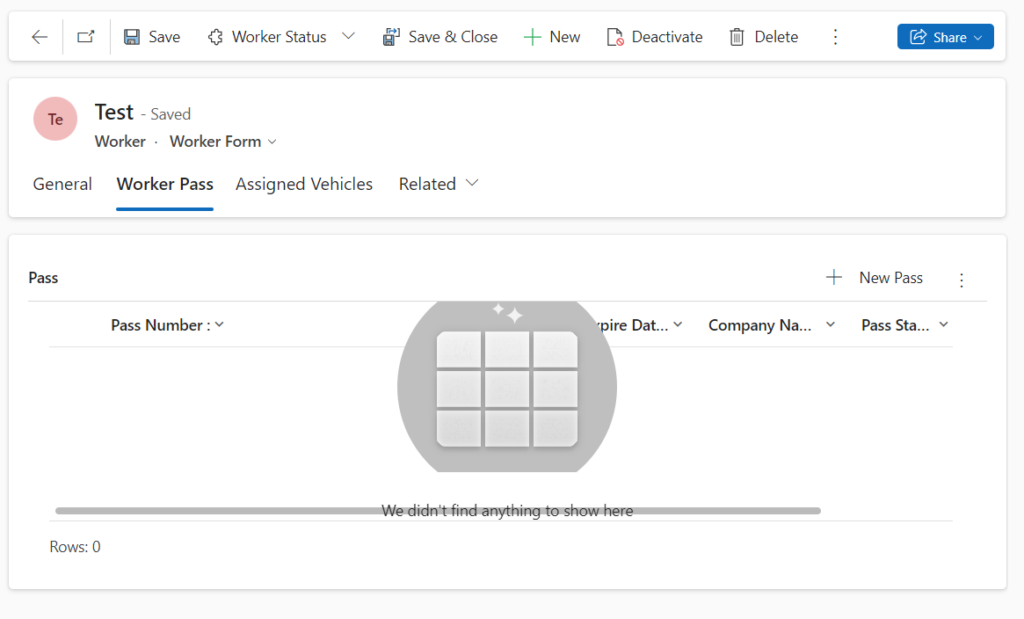
Here, the worker’s status has been approved, and the worker pass given data has been generated.
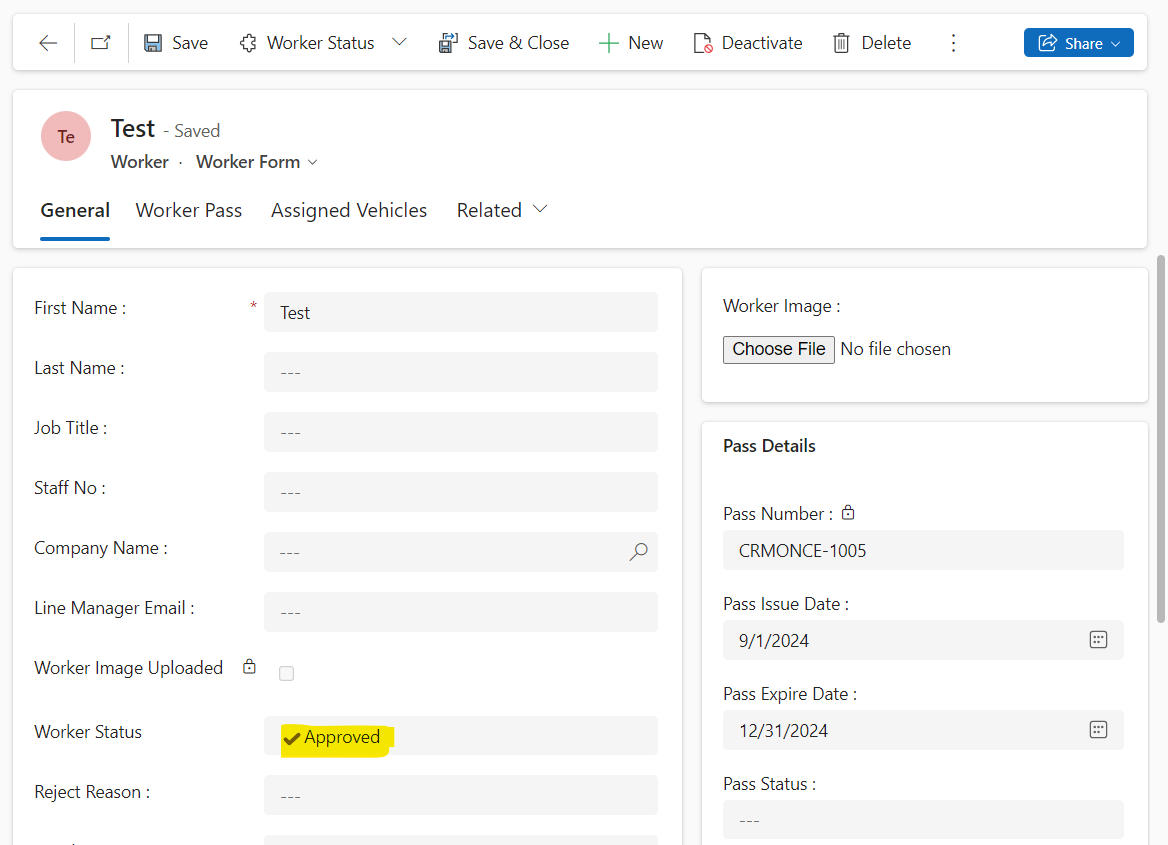
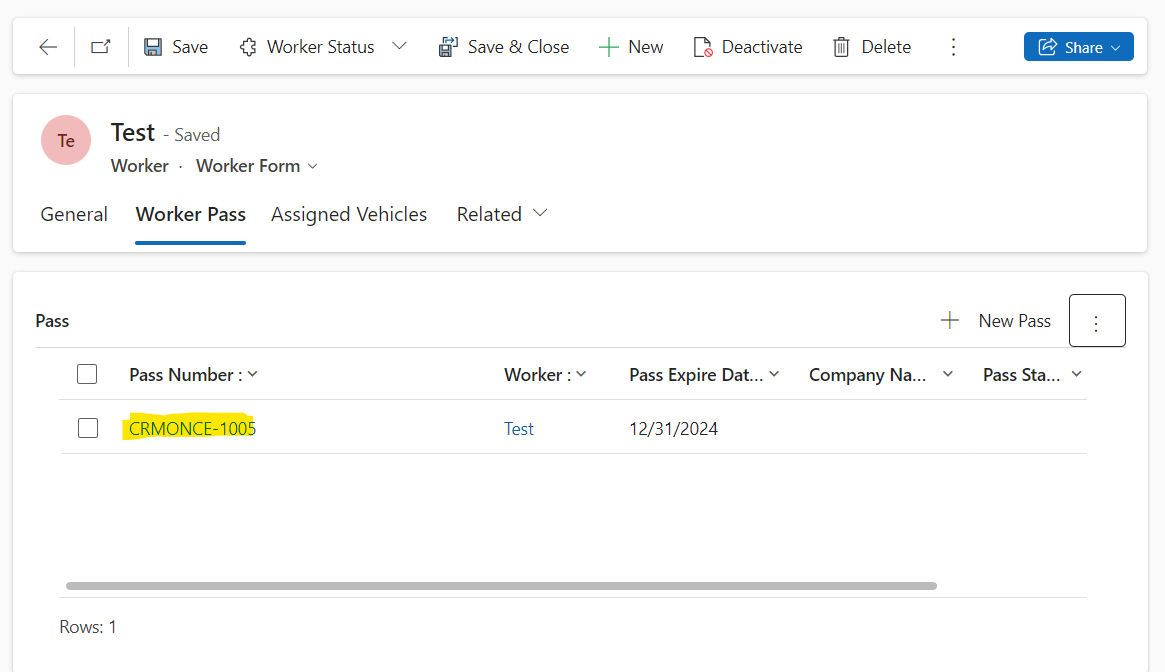
For any Help or Queries Contact us on info@crmonce.com or +91 8096556344