Pass Generate:
The provided code is a Microsoft Dynamics CRM plugin that automatically generates a random passcode when a specific condition is met. It checks if the target entity contains an OptionSet field (test_optionset
) and verifies if its value equals 240110000. If true, it generates a random 8-character alphanumeric passcode and creates a new related entity record, storing the passcode. It also updates the main entity with this passcode.
This plugin is used to automate the creation and association of passcodes when certain conditions in the CRM system are satisfied.
using System;
using System.Linq;
using Microsoft.Xrm.Sdk;
public class PassCodeGeneratorPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
// Obtain the execution context from the service provider.
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
// Obtain the organization service.
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
// Check if the target entity (main entity) is available
if (context.InputParameters.Contains(“Target”) && context.InputParameters[“Target”] is Entity)
{
Entity targetEntity = (Entity)context.InputParameters[“Target”];
// Check if the optionset field is present and valid
if (targetEntity.Contains(“test_optionset”))
{
var optionset = ((OptionSetValue)targetEntity.Attributes[“test_optionset”]).Value;
// If the optionset is set to the value for approval (e.g., 240110000)
if (optionset == 240110000)
{
// Generate a pass number (customizable)
string passNumber = GeneratePassNumber();
// Create a new record in the related entity (Pass Information or Subgrid Entity)
Entity passEntity = new Entity(“test_necn”); // Replace with your subgrid entity logical name
passEntity[“test_name”] = passNumber; // Auto-generated pass number field
// Set the lookup field to the main entity (bank entity)
passEntity[“test_banklookp”] = new EntityReference(targetEntity.LogicalName, targetEntity.Id); // Lookup to the main entity
// Optionally, populate additional fields in the subgrid entity
//passEntity[“test_dateissu”] = DateTime.Now; // Example date field
// Create the record in the subgrid entity
service.Create(passEntity);
// Optionally, update the main entity with the generated pass number
targetEntity[“test_passnumber”] = passNumber; // Update the main entity with pass number
service.Update(targetEntity); // Save the updated main entity record
}
}
}
}
// Method to generate a random pass number
private string GeneratePassNumber()
{
Random random = new Random();
const string chars = “ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789”;
return new string(Enumerable.Repeat(chars, 8) // Customize the length of the pass number
.Select(s => s[random.Next(s.Length)]).ToArray());
}
}
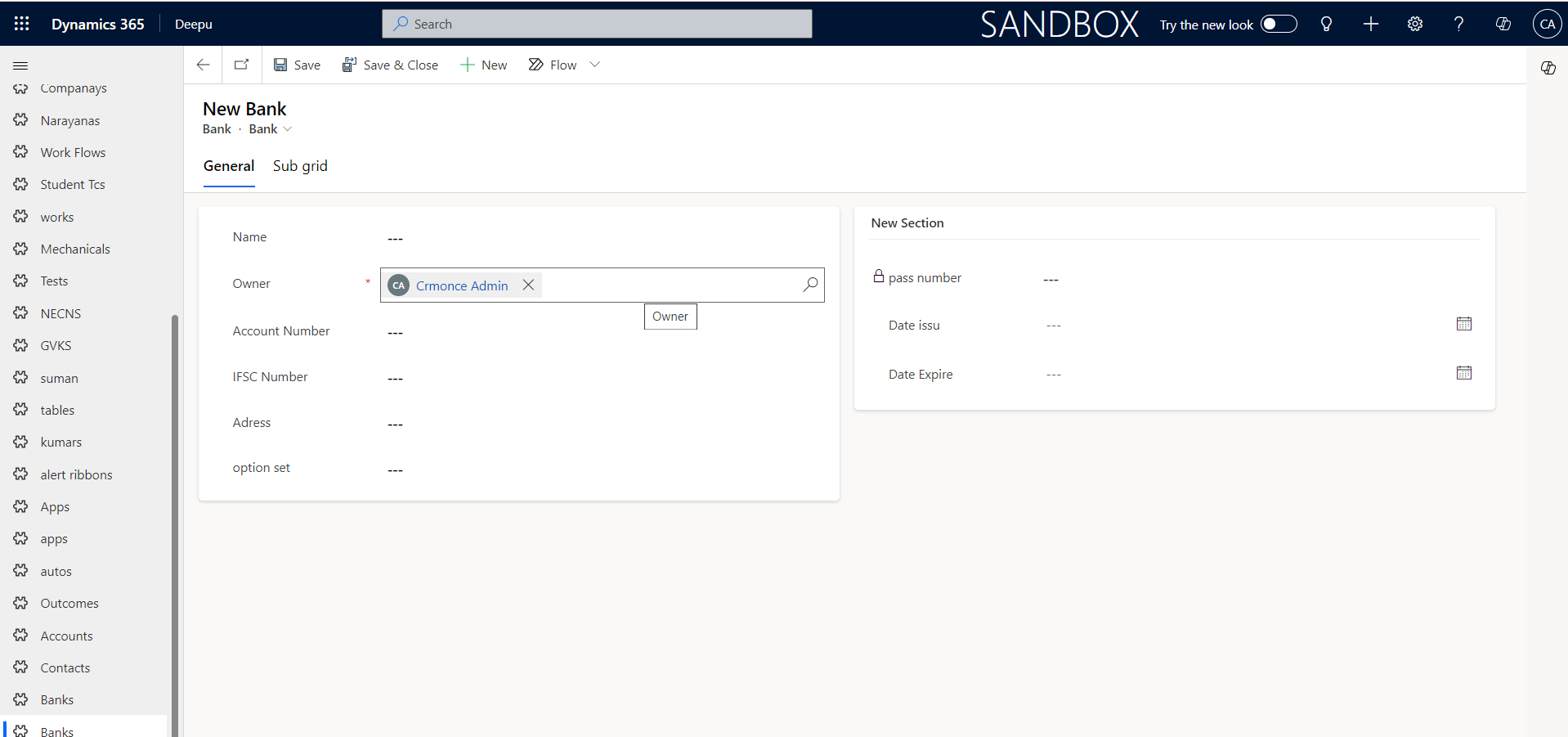
In Microsoft Dynamics 365 CRM form for a “Bank” entity record, named pradeep, in the process of being created. The form contains the following key fields:
- Owner: Set to “Crmonce Admin.”
- Account Number, IFSC Number, Address: These fields are currently blank.
- Option Set: Set to “approved.”
In the New Section, fields for Pass number, Date issu, and Date Expire are displayed but are currently empty. This section likely relates to auto-generated information based on the approval status.
This form is part of the “Banks” entity and is unsaved.
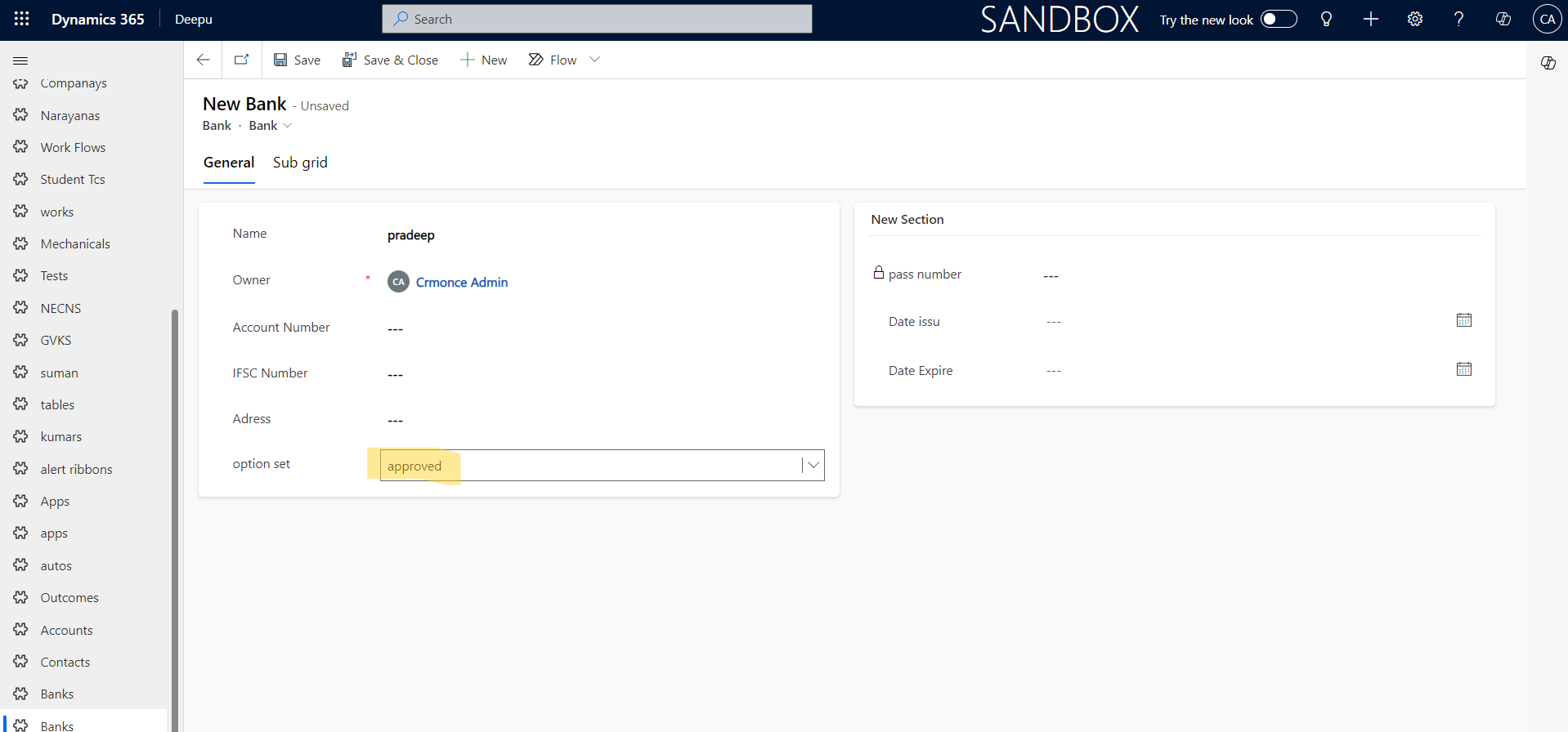
If the option set value is approved, a pass number will be generated Automatically.
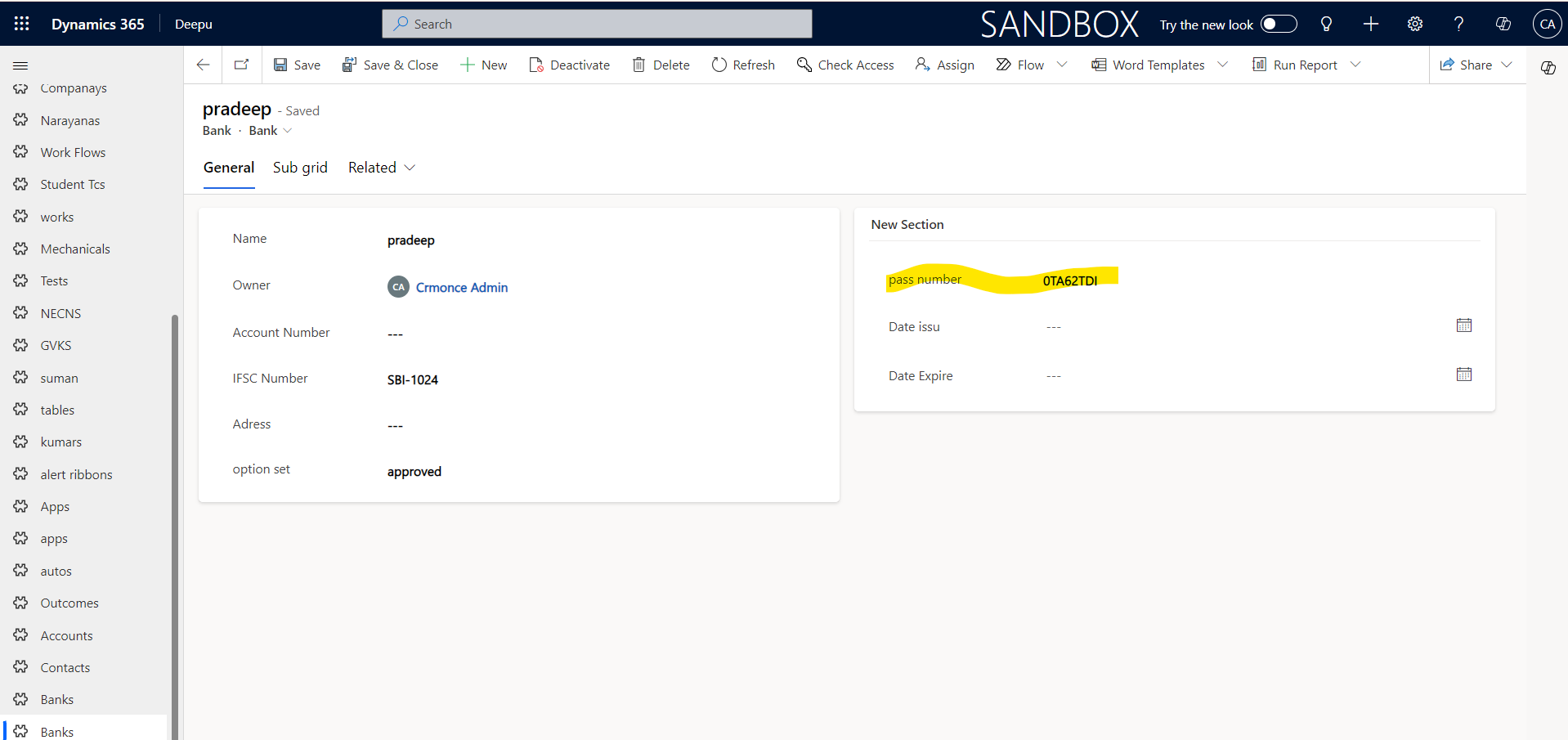
Here, the pass number is generated, and a sub grid gets created automatically.
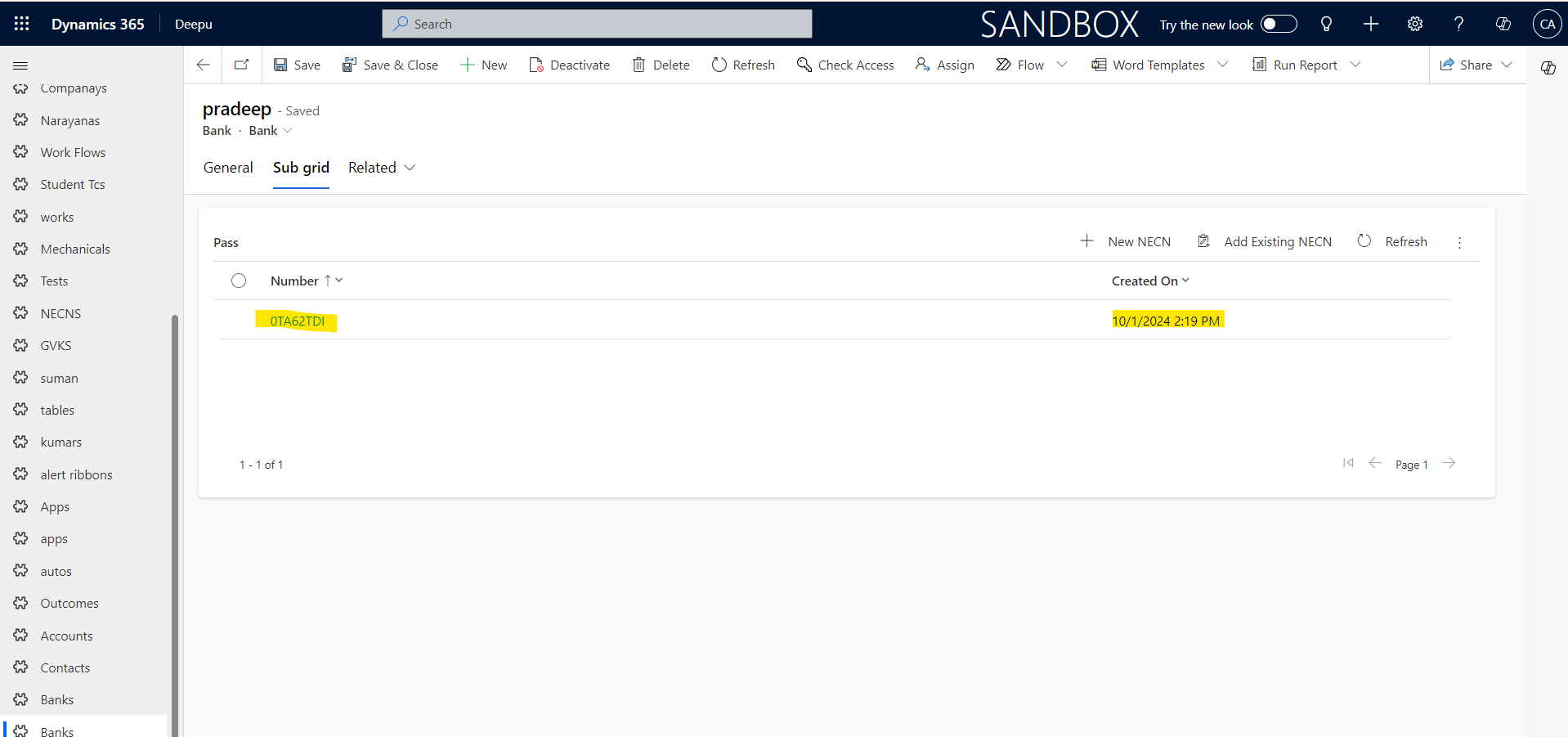
In this case, choose the sub grid record, Then pass number and the lookup value will be generated automatically.
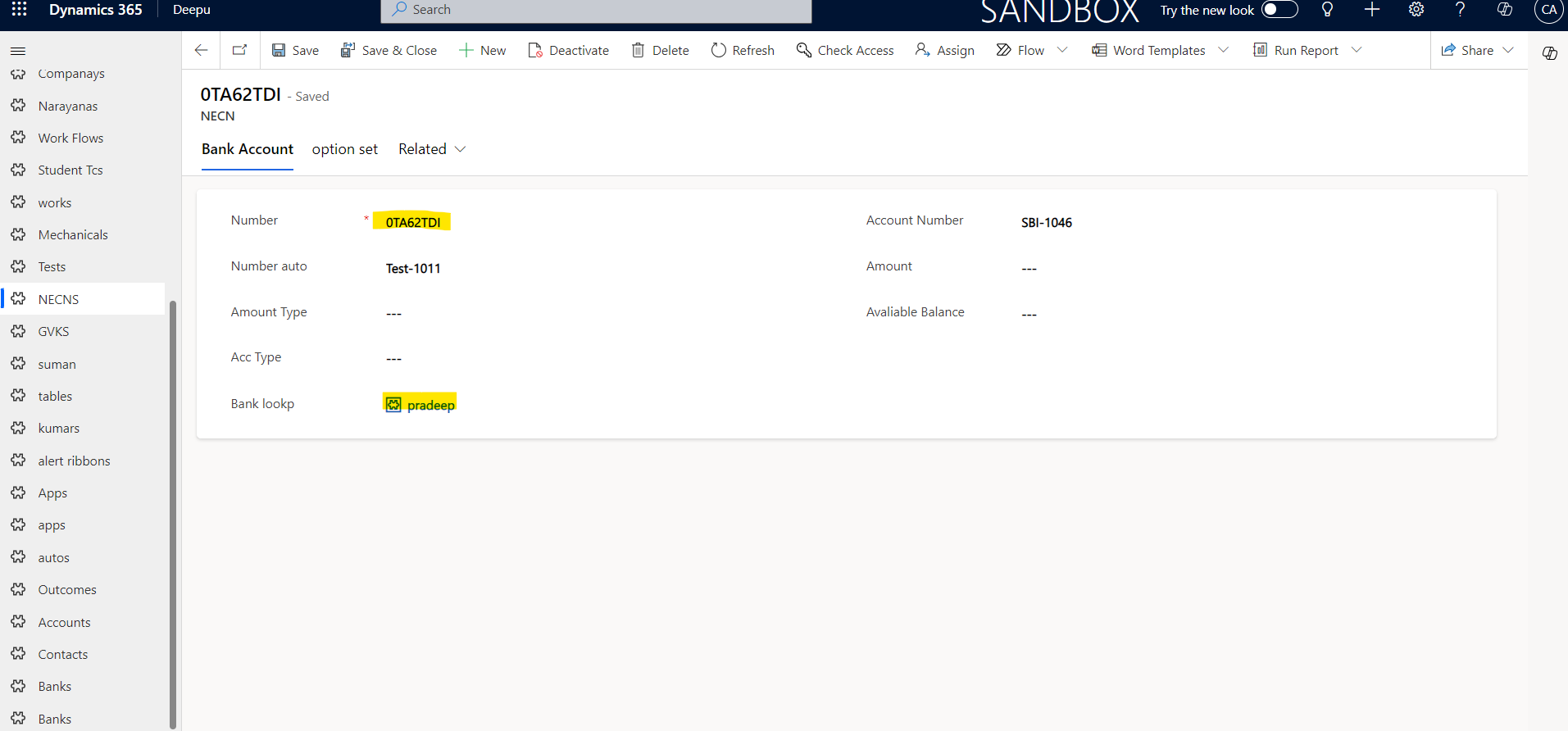
https://www.crmonce.com/field-parameter-in-power-bi-desktop/
For any Help or Queries Contact us on info@crmonce.com or +91 8096556344