Plugin:
In this blog, we will learn about the Basics of plugin
A plugin in Dynamics 365 is custom code that executes in response to specific events in the system (like record creation, update, or deletion). It allows developers to add business logic to the CRM platform. Plugins are executed on the server-side and can be triggered in response to an event in the system.
C# and .NET Basics
To develop plugins in Dynamics 365, understanding C# and the .NET framework is crucial since plugins are written in C#
Object-Oriented Programming (OOP): OOP is a programming paradigm that uses objects and classes to structure software.
It includes concepts like:
Encapsulation: Bundling data and methods that operate on that data.
Inheritance: Creating new classes based on existing classes.
Polymorphism: Methods or classes that can take multiple forms.
Abstraction: Hiding complex implementation details and exposing only the necessary parts.
Interfaces & Dependency Injection:
Interfaces: An interface defines a contract that classes must follow. It helps in achieving loose coupling and enhances testability.
Dependency Injection: This is a design pattern used to implement IoC (Inversion of Control). It makes the system more modular and easier to test.
Exception Handling: C# provides a mechanism to handle runtime errors using try-catch
blocks. This allows you to catch and handle exceptions gracefully.
try
{
// code that may throw an exception
}
catch (Exception ex)
{
// code to handle the exception
}
Dynamics 365 Plugin Development
Plugin Execution Pipeline
The Plugin Execution Pipeline consists of several stages where plugins can be executed. The main stages are:
Pre-Validation:
The plugin executes before any validation or security checks on the data.
Pre-Operation:
The plugin executes before the operation on the data (e.g., create/update).
Main Operation:
The actual operation (e.g., create, update, delete) is performed by the system.
Post-Operation:
The plugin executes after the operation on the data is completed.
The plugins can execute either synchronously (blocking) or asynchronously (non-blocking).
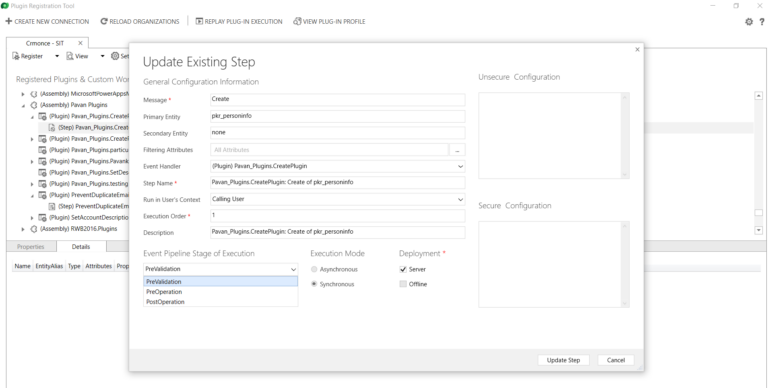
Differences Between Plugin, Workflows, JavaScript, and Power Automate
Plugin:
Server-side logic that executes on events triggered in Dynamics 365.
Written in C#.
Runs in the background when the event occurs.
Allows for complex business logic and integration with external systems.
Trigger: Events like record create/update/delete.
Execution Pipeline: Plugins can run in the pre-operation and post-operation stages.
1. Workflow:
Server-side process in Dynamics 365 that automates business processes.
Configured using the Dynamics 365 interface, no coding required.
Ideal for simple logic like creating tasks or sending emails.
Can be triggered manually or automatically.
Does not have the full power of a plugin (e.g., no direct access to external systems).
2. JavaScript:
Client-side scripting that runs in the user’s browser when interacting with the web interface.
Used to validate data, manipulate forms, and create a better user experience.
It doesn’t have the same capabilities as a plugin because it doesn’t have access to server-side operations.
Trigger: Events like form load, field change, etc.
3. Power Automate:
A cloud-based service that allows users to automate workflows between different applications and services.
Can integrate with various third-party applications (e.g., SharePoint, Outlook, Dynamics 365).
Trigger: Based on events, data changes, or manual initiation.
Low-code/no-code solution that is easy for non-developers to set up and automate workflows.
Debugging Plugins:
You can debug plugins by attaching a debugger to the plugin execution process or using tracing inside the plugin code. In Dynamics 365, use the ITracingService to log debug information that can be viewed in the plugin registration tool.
Alternatively, debugging can be done locally on a test environment by attaching Visual Studio to the process.
Common Data Service (CDS), now called Dataverse, is the underlying data platform for Dynamics 365, Power Apps, and Power Automate. It provides a unified, scalable, and secure data storage and management service for business data across multiple applications.
CRUD Operations (Create, Read, Update, Delete) in Dataverse:
These operations are fundamental for manipulating data within Dataverse. You can perform these actions using the IOrganizationService.
IOrganizationService in C#
The IOrganizationService interface allows you to perform CRUD operations on data stored in Dataverse (formerly CDS). Here’s an example of how you can use IOrganizationService
to perform basic CRUD operations.
using Microsoft.Xrm.Sdk;
public class DataverseExample
{
private readonly IOrganizationService _service;
public DataverseExample(IOrganizationService service)
{
_service = service;
}
// Create operation
public void CreateRecord()
{
Entity contact = new Entity(“contact”);
contact[“firstname”] = “John”;
contact[“lastname”] = “Doe”;
_service.Create(contact);
}
// Read operation
public Entity ReadRecord(Guid contactId)
{
return _service.Retrieve(“contact”, contactId, new ColumnSet(“firstname”, “lastname”));
}
// Update operation
public void UpdateRecord(Guid contactId)
{
Entity contact = new Entity(“contact”, contactId);
contact[“lastname”] = “Smith”;
_service.Update(contact);
}
// Delete operation
public void DeleteRecord(Guid contactId)
{
_service.Delete(“contact”, contactId);
}
}
In this example:
Create
: Creates a new record (e.g., a new contact).
Read
: Retrieves an existing record by its ID.
Update
: Updates an existing record.
Delete
: Deletes a record.
// Create
Entity newAccount = new Entity(“account”);
newAccount[“name”] = “New Account Name”;
Guid accountId = _service.Create(newAccount);
// Read
Entity account = _service.Retrieve(“account”, accountId, new ColumnSet(“name”));
Console.WriteLine(account[“name”]);
// Update
account[“name”] = “Updated Account Name”;
_service.Update(account);
// Delete
_service.Delete(“account”, accountId);
For any Help or Queries Contact us on info@crmonce.com or +91 9493926112.